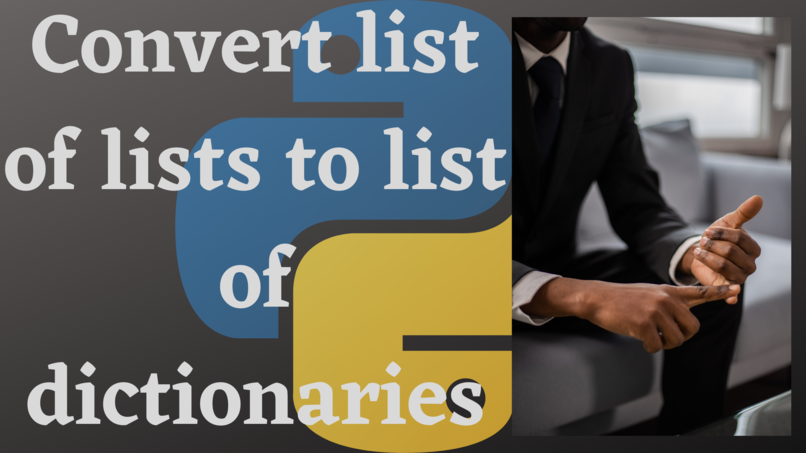
Convert list of lists to list of dictionaries in Python
In the previous article, we have discussed how to convert a list of dictionaries into a list of lists. In this article let’s discuss how to do the converse of this i.e. learn how to convert a list of lists into a list of dictionaries when a separate list that has the data of keys is given to us.
Let us first understand this scenario with an example. We have a list named list_of_list with the following elements.
Example:
list_of_list= [['One', 'Two', 'Three'], ['Two', 'Three', 'Four']]
Output:
We have another list named dict_keys which has the keys for the list of dictionaries that we are creating.
Example:
dict_keys = [1,2,3]
Output;
We now have to get the output in this form which is a list of dictionaries.
Example:
[{1: 'One', 2: 'Two', 3: 'Three'}, {1: 'Two', 2: 'Three', 3: 'Four'}]
Output:
We can achieve this using two methods:
- Using list comprehension
- Using dictionary comprehension
Before we learn these methods, having prior knowledge about list comprehension and dictionary comprehension is appreciated. So please refer to the articles below
list comprehension
dictionary comprehension
Using list comprehension:
We are using the dict() function along with the zip() function which will zip the values of elements in the list dict_keys and the lists in the list_of_list and store them in a list named list_of_dict.
[ dict (zip(dict_keys, i)) for i in list_of_list ]
Example:
list_of_list= [['One', 'Two', 'Three'], ['Two', 'Three', 'Four']] dict_keys = [1,2,3] print("Given list of lists: " +str(list_of_lists)) print("The given keys are: " +str(dict_keys)) list_of_dict = [dict(zip(dict_keys, i)) for i in list_of_list ] print("The given keys are: " +str(list_of_dict))
Output:
Using dictionary comprehension:
The implementation is much similar to that of list comprehension. We use a zip() function here which again does the same task as above in the one-liner code using dictionary comprehension and stores it in a list named list_of_dict.
list_of_dict = [{ k:v for k,v in zip(dict_keys, i) } for i in list_of_list]
Example:
list_of_list= [['One', 'Two', 'Three'], ['Two', 'Three', 'Four']] dict_keys = [1,2,3] print("Given list of lists: " +str(list_of_lists)) print("The given keys are: " +str(dict_keys)) list_of_dict = [{k:v for k,v in zip(dict_keys, i)} for i in list_of_list ] print("The converted list: " +str(list_of_dict))
Output: