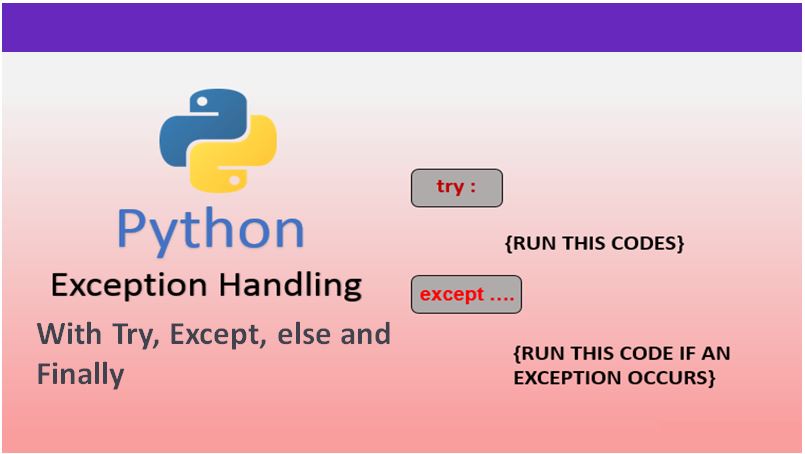
Exception handling with try, except, else and finally in Python
During the execution of the program an exception might occur which is also known as run time error. Python generates an exception during the execution when that error occurs and it can be handled, that avoids your program to interrupt.
Example:
a = 5 b = 0 print(a/b)
Output:
In above example code, the system cannot divide the number with zero then an exception is raised.
Exception handling with try, except, else and finally
- Try: This block will check for the excepted error to occur.
- Except: Here you could handle the error
- Else: If no exception occurs then this block will be executed.
- Finally: Finally block always be executed either exception occurs or not
Let’s understand how the try and except works –
- Initially try clause will be executed
- If there is no exception, then try clause will execute and except clause is over.
- If there is any exception generated, the try clause will be skipped and except clause will execute.
- If any exception occurs, but the except clause within the code could not handle it, it is passed on to the outer try If the exception is not handled, then the execution stops.
- A try statement may have one or more except clause
Example:
def divide(x, y): try: # Floor Division : Gives only Fractional # Part as Answer result = x // y print("Yeah ! Your answer is :", result) except ZeroDivisionError: print("Sorry ! You are dividing by zero ") # parameters passing and note the working of Program divide(8, 4) divide(5, 0)
Output:
Else Clause
The program will enter into the else block only if the try clause do not generate an exception.
Example:
def divide(x, y): try: # Floor Division : Gives only Fractional # Part as Answer result = x // y except ZeroDivisionError: print("Sorry ! You are dividing by zero ") else: print("Yeah ! Your answer is :", result) # Look at parameters and note the working of Program divide(8, 4) divide(5, 0)
Output:
Finally Keyword
Finally keyword is provided by Python, which is always executed after try and except blocks.
Example:
Let’s try to throw the exception in except block and Finally will execute even though exception generates or not.
def divide(x, y): try: # Floor Division : Gives only Fractional # Part as Answer result = x // y except ZeroDivisionError: print("Sorry ! You are dividing by zero ") else: print("Yeah ! Your answer is :", result) finally: # this block is always executed # regardless of exception generation. print('This is always executed') # Look at parameters and note the working of Program divide(8, 4) divide(5, 0)
Output:
Share: