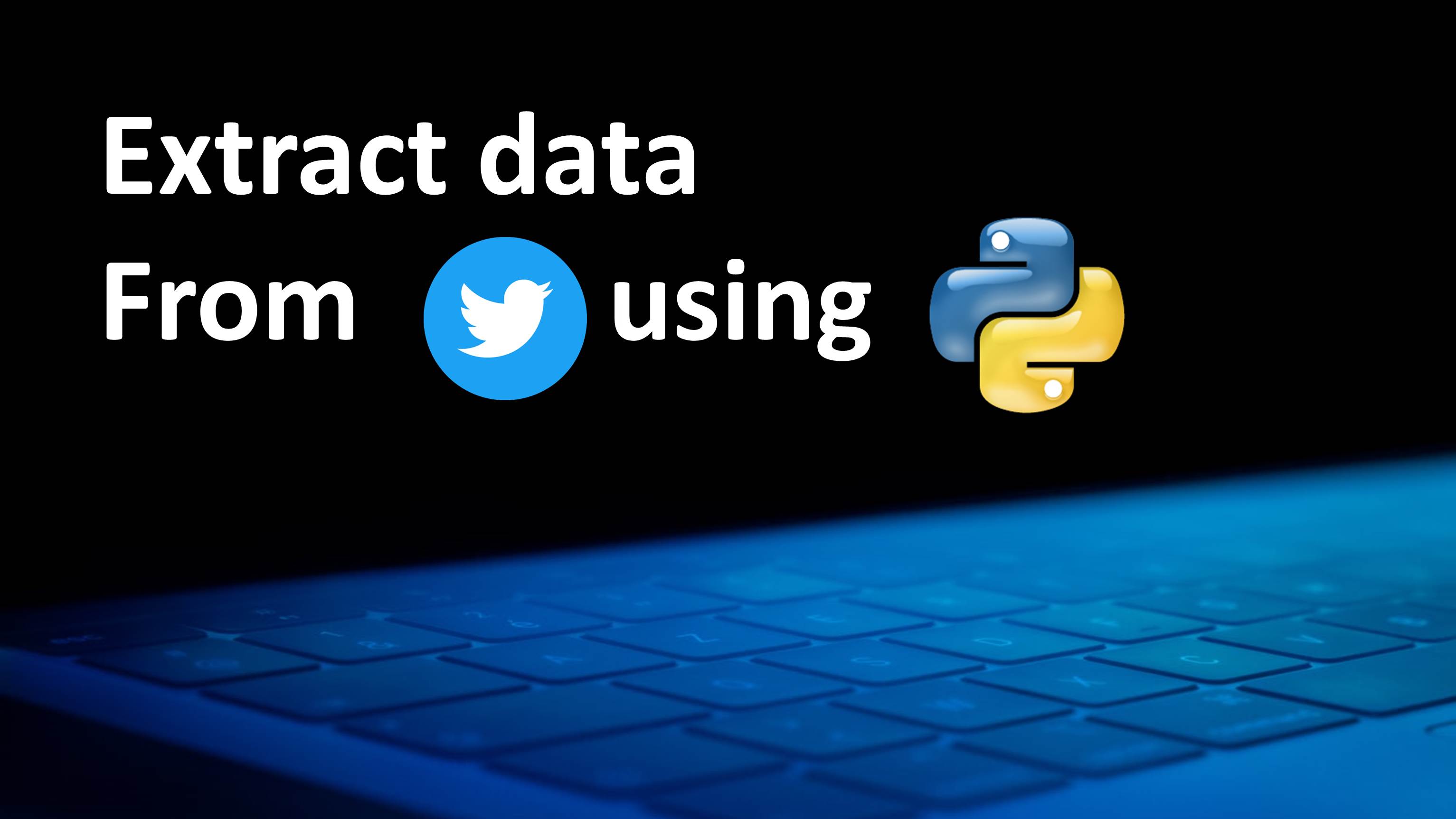
Extract data using Twitter API and python
In this article, we are going to extract the data using twitter API ( Application programming interface )
Twitter is one of the social networking website where users can post and interact with messages known as tweets. Twitter can help to mine the data from the user twitter account using the Twitter API or Tweepy.
What is Tweepy?
Python has packages that can interact with the Twitter account. One of the most useful Python Twitter packages is Tweepy, It can give access to the Twitter API. It can expose the tweets, retweets,
Likes, direct messages, and Followers. The data is extracted from the user tweet.
Getting started :
The first step is to get the consumer key, consumer secret, access secret from twitter developer made easily available for each user. This key is for authentication of API.
To get these keys first create the twitter account is you don’t have the Twitter account for getting the credentials we will need to access Twitter. To do that we will have to apply for the developer account.
The official website to apply the developer account is given below :
https://developer.twitter.com/en
For obtaining the keys you need to follow some steps given below:
- Login to the twitter developer section
- Go to create an app
- Fill the details of the application and click on create your twitter application
- After creating your twitter application details of the application will be shown along with consumer key and consumer secret.
- For accessing the token, click on “Create my access token “. The page will generate an access token.
By following the above steps we will generate our consumer key, consumer secret, access secret.
Next we will have to install the Tweepy python libraries . This can be accomplished by using pip
Code :
pip install tweepy
By this we have installed the tweepy or twitter API now we can start using it . we need to use the OAuth Interface to authorize our app to access Twitter
To extract the data of tweets by using tweepy python library code is given below :
import tweepy # Fill the X's with the credentials obtained by # following the above mentioned procedure. consumer_key = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" consumer_secret = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" access_key = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" access_secret = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" # Function to extract tweets def get_tweets(username): # Authorization to consumer key and consumer secret auth = tweepy.OAuthHandler(consumer_key,consumer_secret) # Access to user's access key and access secret auth.set_access_token(access_key, access_secret) # Calling api api = tweepy.API(auth) # 200 tweets to be extracted number_of_tweets=200 tweets = api.user_timeline(screen_name=username) # Empty Array tmp=[] # create array of tweet information: username, # tweet id, date/time, text tweets_for_csv = [tweet.text for tweet in tweets] # here CSV file created for j in tweets_for_csv: # Appending tweets to the empty array tmp tmp.append(j) # Printing the tweets print(tmp) # Driver code if __name__ == '__main__': # Here is the twitter handle for the user # whose tweets are to be extracted. get_tweets("twitter-handle")
From above mention code the CSV file is generated .The CSV file contain the data of tweets .
In the above code we have used the following function :
OAuthHandler(): From this object, we will log in to Twitter
set_access_token() : this function is used for setting the access the token
API(): you can create this API() instance that will allow you to access Twitter.
In this way, we have the extract data using Twitter API and python.