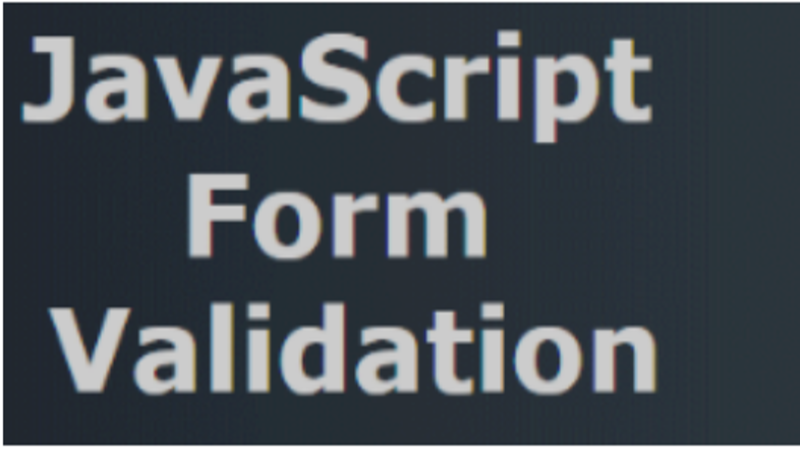
Form Validations using JavaScript
The user-submitted form has to be validated because it may contain values that are incorrect. In order to authenticate a user, validation is necessary. Because JavaScript allows form validation on the client side, data processing is faster than server-side validation. JavaScript form validation is preferred by the majority of web developers.
We can validate name, password, email, date, mobile numbers, and other fields using JavaScript.
In this example, we’ll validate the name and password. The name cannot be blank, and the password cannot be less than six characters long.
JavaScript Code:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <title>Form Validations using JavaScript</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="validate.css"> </head> <body> <script> function validateform() { var name = document.myform.name.value; var password = document.myform.password.value; if (name == null || name == "") { alert("Name can't be blank"); return false; } else if (password.length < 6) { alert("Password must be at least 6 characters long."); return false; } } </script> <div id="error"> <form class="form" name="myform" method="get" onsubmit="return validateform()"> <!-- <fieldset> --> <ul> <li><label for="name">Name</label></li> <li> <input type="text" name="name" id="name"></li> <li><label for="password">Password</label></li> <li> <input type="password" name="password" id="password"></label></li> <input class="btn" type="submit" value="Form Submit"> </ul> <!-- </fieldset> --> </form> </div> </body> </html>
In the above code we are using if else conditioning blocks to validate user input. When the specified conditions are not met it throws an alert pop up on the webpage to notify the user on their invalid form submission.
Validate.css
*{ margin: 0px; padding:0px; } body{ background-image: url('validate.jpg'); background-repeat: no-repeat; width:100%; background-size: cover; } ul{ margin-left:400px; border: 1px solid rgb(171, 223, 898); width:400px; height: 270px; margin-top: 140px; } ul li{ list-style-type: none; margin-left: 25%; padding: 4px; margin-top: 10px; } .btn{ background-color:rgb(10, 245, 10); color: aliceblue; padding: 5px; margin-left: 41%; margin-top: 18px; } .form{ background-image: url('validate.jpg'); background-repeat: no-repeat; width:100%; background-size: cover; }
On form submission, we validate the form. The user will not be taken to the next page unless the values entered are correct.
Output: