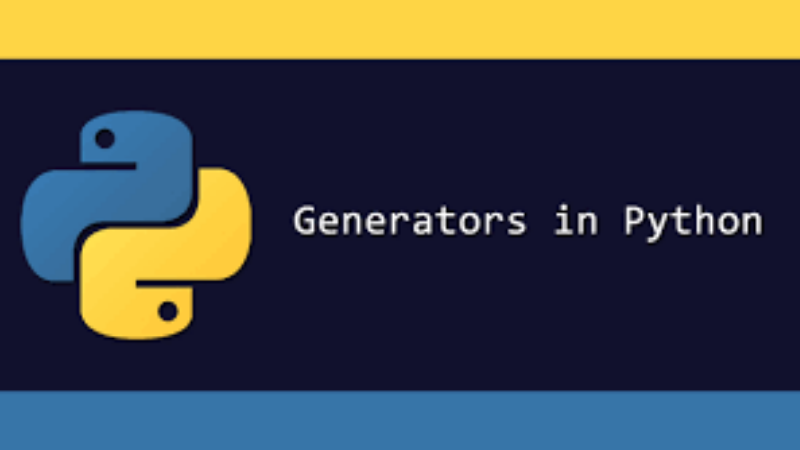
Generators in Python
Generator-Function: A generator-function is a special type of function which is defined like a normal function, but it returns or generates a value using the yield keyword rather than using return. If any function contains the yield keyword in its definition, the function automatically becomes a generator function. Generator functions contain one or more yield statements. Unlike a normal function which returns a single value, the generator function returns an iterator object with a sequence of values.
The generator function, when called, does not start execution immediately, it returns an object (iterator). Here, we iterate through the elements in the sequence using next() and methods __next__() and __iter__() are implemented automatically. Then, the function pauses and the control goes to the caller once the function yields. Finally, StopIteration gets activated automatically once the function gets terminated for further calls.
Example:
def fun(): n = 0 print('First statement') yield n n += 1 print('Second statement') yield n n += 1 print('Third statement') yield n for i in fun(): print(i)
output:
When we use the yield keyword to return data from a function, it starts storing the states of the local variable, as a result, the overhead of memory allocation for the variable in consecutive calls is saved. Also, as the old state is retained in consecutive calls, the flow starts from the last yield statement executed, which in turn, saves time.
Note: The generator object can be iterated only once and must create another generator object like a = fun() to restart the process. It’s because a for loop uses the next() function to iterate over an iterator, here ‘i’. And when StopIteration gets activated, it ends automatically.
Generally, the generator functions are implemented using a loop like in the example below.
Example:
def fun(a): length = len(a) for i in range(length -1, -1, -1): yield a[i] #For loop to reverse the string for i in fun1("i2tutorials"): print(i)
output:
This is an example of a generator that reverses a string. Here, we used the range() function which gives the string back in reverse order with the help of a for loop.
Note: We can also use other kinds of iterables like list, tuple, etc other than strings in this generator function.