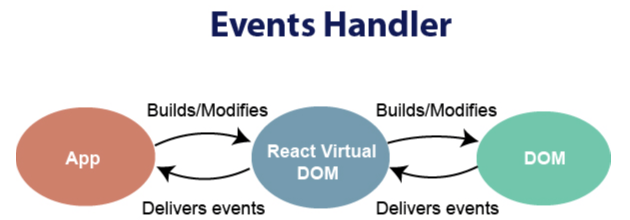
Getting started with React SyntheticEvent
With React event SyntheticEvent, we can access the react event payload. In the form input field, we define an onChange callback function, as shown below:
/ src/App.js/
class App extends Component { // Some piece of codes... render() { return ( <div className="App"> <form> <input type="text" onChange={this.onSearchChange} /> </form> // Some piece of codes... </div> ); } }
Next, we will bind and define the event method; the function() is bound to the component, and it is a class method:
// src/App.js/
class App extends Component { constructor(props) { super(props); this.state = [ list, ]; this.onSearchChange = this.onSearchChange.bind(this); this.onDismiss = this.onDismiss.bind(this); } onSearchChange(){ // Some piece of codes } // Some piece of codes... }
With the method argument, we can now have access to the synthetic React event. The event now has the value of the form input field and the event payload. Essentially,e is a synthetic react event, giving us the ability to changing the state of searchName, as shown below:
// src/App.js/
class App extends Component { // Some piece of codes onSearchChange(e){ this.setState({ searchName: e.target.value }); } // Some piece of codes... }
We need to give searchName an react initial state in the constructor, as shown below:
class App extends Component { constructor(props) { super(props); this.state = [ list, searchName: '', ]; this.onSearchChange = this.onSearchChange.bind(this); this.onDismiss = this.onDismiss.bind(this); } // Some piece of codes... }
Share: