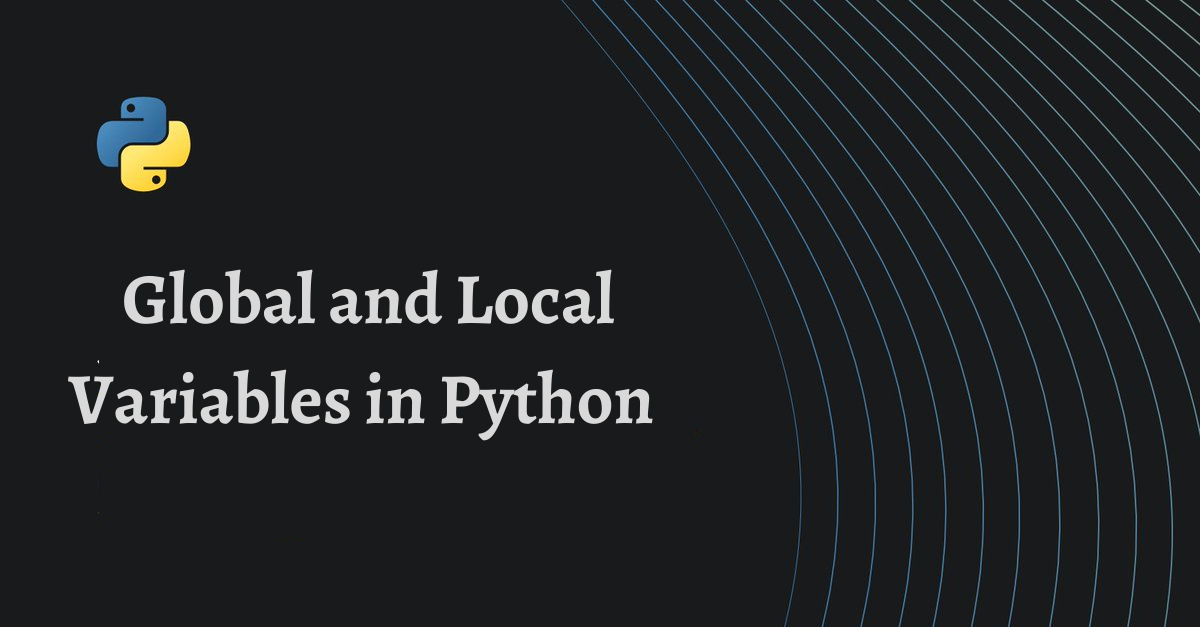
Global and Local Variables in Python
Global Variable:
A variable defined and declared outside a function or in global scope is known as a global variable. Such a variable can be accessed inside or outside the function but can be used inside a function.
Example:
a = "I am global" def i_am_global(): print("a in the function:", a) i_am_global() print("a in the function:", a)""
Output:
In the example code, we have created a global variable a and defined a function i_am_global() to print the global variable ‘a’. And again, we call the function i_am_global(), which will again print the value of ‘a’.
Is there any possibility to change the value of ‘a’ inside a function?
Example:
p = "I am global" def fun(): p = p * 2 print(x) fun()
Output:
As we can see, we get a UnboundLocalError because Python is treating p as a local variable and p is also not defined inside fun().
Now to solve this, Python has come up with a new keyword named “global”.
The global keyword allows us to change the value of the variable outside of the present scope. It allows us to create a global variable and makes changes to the variable in a local context.
Example:
d = 10 def function(): global d d = d * 2 print("Inside function():", d) function() print("In main:", d)
Output:
In the example code, we defined d as a global keyword inside the function() function.
We can see that by using the global keyword change, the global variable d too, outside the function, i.e. d= 20.
Local variable:
A variable defined and declared inside the function’s body or in the local scope is known as a local variable.
Creating a local variable:
Example:
def fun2 (): y = "I am local" print(y) fun2()
Output:
Accessing Local variables outside their scope:
Example:
def fun1(): l = "local variable" fun1() print(l)
Output:
In the above example code, we face an error named NameError as we’re trying to access a local variable ‘l’, which is in the global scope. In contrast, the local variable only works inside fun1() or local scope.
Using Global and Local variables in the same code
Example:
n = "I am global " def fun3(): global n s = "I am local" n = n * 2 print(n) print(s) fun3()
Output:
We declared a global variable ‘n’ and a local variable ‘s’ in the fun3() function in the above example code. Then, we tried to modify the value of global variable n and print both n and s.
Global variable and Local variable with same name:
Example:
r = 15 def fun4(): r = 10 print("local r:", r) fun4() print("global r:", r)
Output:
In the above example code, we used the same variable name r for both global variable and local variable. As the variable is declared in both scopes, i.e. the local scope inside fun4() and global scope outside fun4(), even though we print the same variable we get different outputs.
The output is local r:10 when we print the variable inside fun4(). This is the local scope of the variable.
And the output is global r:15 when we print the variable outside the fun4(), this is the global scope of the variable.