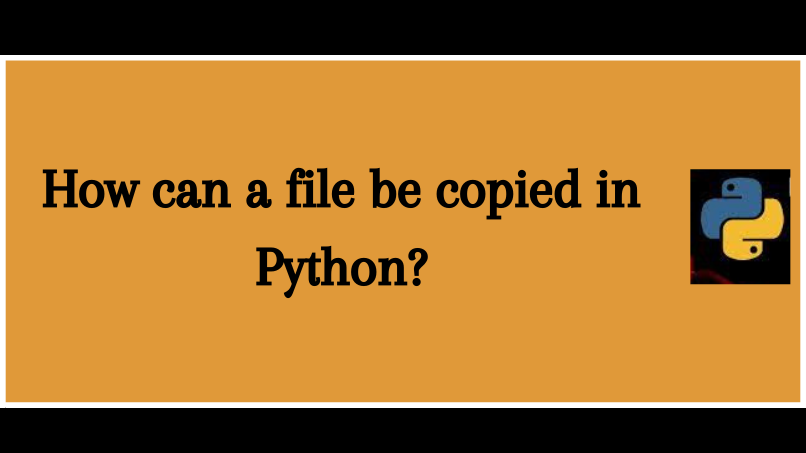
How can a file be copied in Python? Part- I
Using the shutil module
Python is enabled with the shutil module which has high-level methods to copy files. It comes under Python’s standard utility modules. All the methods this module supports are mentioned below.
- Using copyfile()
- Using copy()
- Using copy2()
- Using copyfileobject()
Let’s discuss each of these methods in detail.
1. Using copyfile():
This method copies all the contents from the source file to the destination file. It takes in the following parameters.
Example:
shutil.copyfile(src_file, dest_file, *, follow_symlinks=True)
Output:
src_file is a string that represents the path of the source file.
dest_file is a string that represents the path of the destination file.
follow_symlinks is an optional parameter that by default is set to the boolean value True and if it is set to False and the source file is a symbolic link then instead of copying the contents to a new file, a symbolic link is created.
* specifies that all the parameters here are keyword-only parameters, here follow_symlinks.
Note: A symbolic link or a symlink is a term for any file that contains a reference to another file or directory in the form of an absolute or relative path.
Example:
import shutil shutil.copyfile('source_file.txt', 'destination_file.txt')
Output:
Here we tried copying contents from our source file named source_file.txt to the destination file destination_file.txt.
In this method, the destination file must be writable and the name shouldn’t be the same as the source file else it raises the SameFileError exception. If the destination file already exists, then it gets replaced by the newly copied file.
2. Using copy():
This method is much similar to the above method. But here one extra feature gets added i.e., the file permissions from the source file also get copied to the destination. Copying file permissions is quite an insignificant task in most of programming languages so it’s a good feature to have. The syntax is similar to the copyfile() method.
Example:
import shutil shutil.copy('source_file.txt', 'destination_file.txt')
Output:
3. Using copy2():
This method works similar to the copy() method and also has a similar syntax.
Example:
import shutil shutil.copy('source_file.txt', 'destination_file.txt')
Output:
It performs an additional task i.e., it also preserves the source file’s metadata. For example the creation date of the source file, etc.
Note: A file’s metadata is extra information about a file that is embedded in or associated with the file. Metadata isn’t the actual document or photo itself, it’s the information about it, for example, the file’s name, thumbnail image, or its creation date.
4. Using copyfileobject():
This method copies the content from the source file into a destination file, from the current source-file position i.e. when you read data from the source file object, the position you stop reading at, is the position the copyfileobj() method starts copying from.
The syntax of this copyfileobject() method is
Example:
shutil.copyfileobj(src_file_object, dest_file_object[, length])
Output:
The src_file_object and the dest_file_object are similar to the above-mentioned src_file and dest_file parameters but the difference is that they refer to objects.
The length parameter is optional which represents the buffer size. Buffer size is the number of bytes used/kept in memory during the copy process. This parameter is of great help when we copy large files as it speeds up the copying process and ensures efficient memory usage.