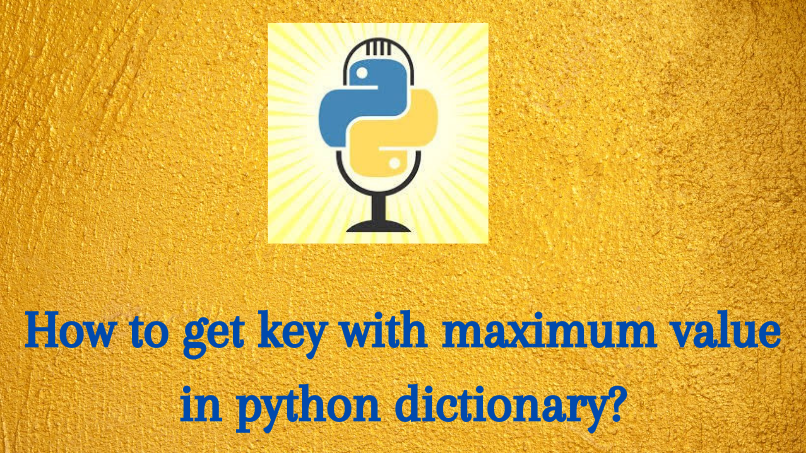
How to get key with maximum value in Python dictionary?
We can find the key with maximum value in a given dictionary using the following ways:
- Using max()
- Using max() with lambda
- Using operator
- Using manual method
Using max():
Syntax:
max(iterable, key, default)
- iterable can be a list, a dictionary, a tuple, etc.
- key performs comparison based on return type of the iterables passed. This is an optional parameter.
- default is the default value to be returned by the max() function in case the iterable is empty.
It returns the maximum value from an iterable.
Example:
dictionary= {'One': 1, 'Two':2, 'Three':3} max_key = max(dictionary, key=dictionary.get) print("The key with maximum value is: ",max_key)
Output:
Using operator:
We first import the operator module. The itemgetter() function returns nth index element of an iterable, be it a list, dictionary, tuple, etc., which it takes in as an input. The items() dictionary method gets all the items i.e. key-value pairs from a dictionary and finally, we use the max() function to get the key with maximum value.
Example:
import operator dictionary= {'One': 1, 'Two':2, 'Three':3} max_key = max(dictionary.items(), key = operator.itemgetter(1))[0] print("The key with maximum value is: ",max_key)
Output:
Using lambda with max():
Lambda functions also referred to as anonymous functions, are regular functions defined without a name. We don’t use def to define these functions, instead we use lambda.
Example:
dictionary= {'One': 1, 'Two':2, 'Three':3} max_key = max(dictionary, key= lamda x: dictionary[x]) print("The key with maximum value is: ",max_key)
Output:
Using manual method:
We define a function named max_key, we get the keys and values of our dictionary using keys() and values() dictionary methods and make them a list using the list() function. We now return an element from the list k whose value v at a particular index has a maximum value using the index() and max() functions.
Example:
dictionary= {'One': 1, 'Two':2, 'Three':3} def max_key(dictionary): v=list(dictionary.values()) k=list(dictionary.keys()) return k[v.index(max(v))] max_key(dictionary)
Output: