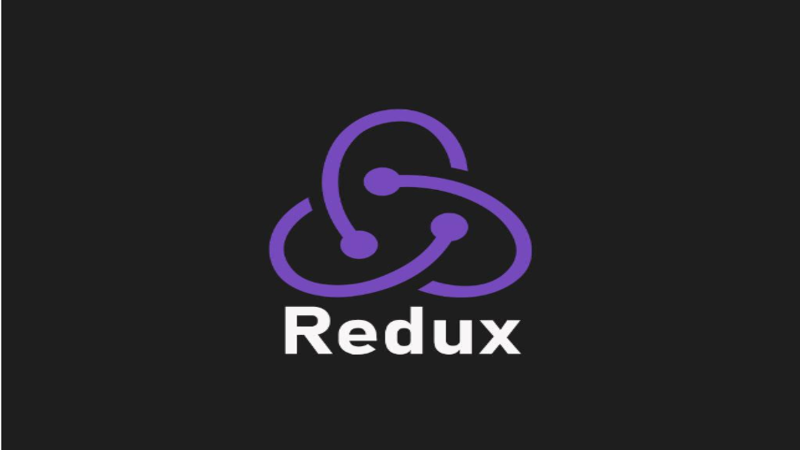
How to properly set initial state in redux?
Table of Contents:
- Initializing State
- createStore Pattern
- Reducer Pattern
- Closing Thoughts
1. Initializing State
In React Redux, all your application state is hold on to in the store; which is an object that holds the complete state tree of your apps. There is only one way to change its react state and that is by react redux dispatching redux actions.
React actions are objects that be made up of a type and a payload property. They are generated and dispatched by special functions called action creators.
see a small contrived and detailed example in the below:
First creating the Redux store:
import { createStore } from 'redux' function todosReducer(state = [], action) { switch (action.type) { case 'ADD_TODO': return state.concat([action.payload]) default: return state } } const store = createStore(todosReducer)
Next updating the store
const ADD_TODO = add_todo; // creates the action type const newTodo = ["blog on dev.to"]; function todoActionCreator (newTodo) { const action = { type: ADD_TODO, payload: newTodo } dispatch(action) }
Like I said my examples are small and bring about and are aimed at clearing the air, around the aspect of react Redux related to our discussion. So, kindly see them as a refresher. I am take it you already have some understanding of react Redux and are familiar with some patterns in a React Redux environment.
However after everything of your professional experience, I would not be surprised if you find a hoe on these premises because too many people know too much but actually know nothing at all.A lot of web developers where making thunderclaps around the web about hoisting, but they were all explaining the concept wrongly.
When a redux store is generated, and React Redux dispatches a dummy redux action to your reducer to populate the store with the beginning state. You are not signify to handle the dummy action directly. Just remember that your react reducer should return some kind of beginning state if the state given to it as the first argument is undefined.
But you do not want your beginning application state to be undefined,and so you have to initialize the state yourself. There are two different ways or patterns to do this viz: the createStore pattern and the reducers pattern.
2. createStore Pattern
The react createStore method can accept an optional preloadedState value as its second argument. In our example, we are called createStore without passing this value. When a value is passed to the preloadedState it becomes the beginning state.
const initialState = [] // in a real-world, is way better to initialize state with {} (an object) instead of an array. const store = createStore(todosReducer, initialState)
let’s say we have a mandatory todo list for each and every one and we can add new tasks to this list later. In this case, we are initialize state like this:
const initialState = ["eat", "code", "sleep"]; // compulsory todo list const store = createStore(todosReducer, initialState)
3. Reducer Pattern
Reducers can also specify an beginning state value by looking for an incoming state argument that is undefined, and/or returning the value they’d like to use as a default. In our example above your todoReducer already does this.
function todosReducer(state = [], action) { switch (action.type) { case 'ADD_TODO': return state.concat([action.payload]) default: return state } }
But there is a drawback to this method. In your contrived example, it can be special but what of in a big application where we have about 10 or 20 react reducers and we want to initialize state not just with an empty array([]) and/or object literal({}), but with some data. It would take a lot of repeating to get this done with the reducers and it is going to be tedious if we decide to change that initial state data at some point.
4. Closing Thoughts:
Reducers whose beginning state is populated make use of preloadedState will still need to provide a default value to handle when passed a react state of undefined. All reducers are passed undefined on initialization state, so they should be written such that when given undefined, some value should be returned. This can be any non-undefined value; there is no need to copy the section of preloadedState here as the default.