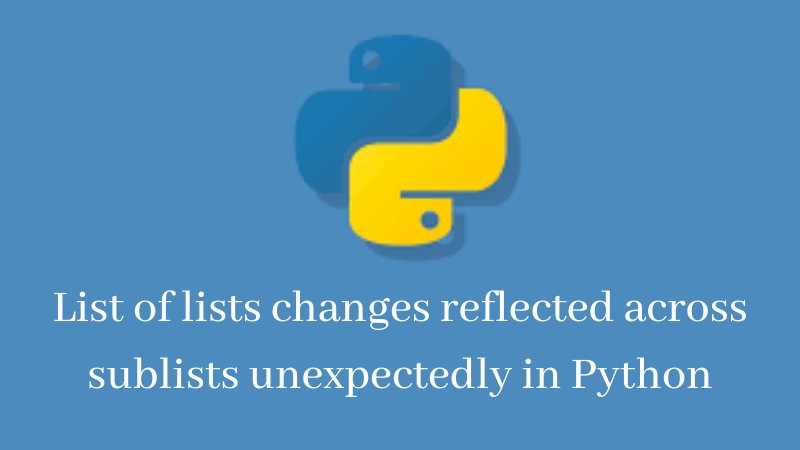
List of lists changes reflected across sublists unexpectedly in Python
Before we dive into the solution, let’s try to understand the question.
We have a list of lists named p which has the following elements in it
p=[2,2,2]
Now we multiply this list into two using the * operator to form two sublists like in the code shown below.
Example:
p=[[2,2,2]]*2 print(p)
Output:
After this modification, we get a list of lists. Now, if we try to change any one particular element in one of the sublists, the change is seen in the others too.
Example:
p=[[2,2,2]]*2 print(p) p[0][1]=3 print(p)
Output:
Why is this happening in the first place?
The * operator simply operates with objects. It just creates references, all pointing to the same object. It doesn’t specifically make a copy or separately evaluate each one of them.
The * operator in this example is just trying to make new references of the existing sublists rather than creating new ones.
How do we prevent this?
By using list comprehension along with the range() function.
Example:
p=[[2]*4 for _ in range(2)] print(p) p[0][1]=3 print(p)
Output:
In this approach, the element gets re-evaluated in every iteration. For every evaluation of this element here [2]*4, it creates a new sublist.
So the change made is reflected not on all sublists but on only one of them.
Note: To learn more about list comprehension refer to the article list comprehension