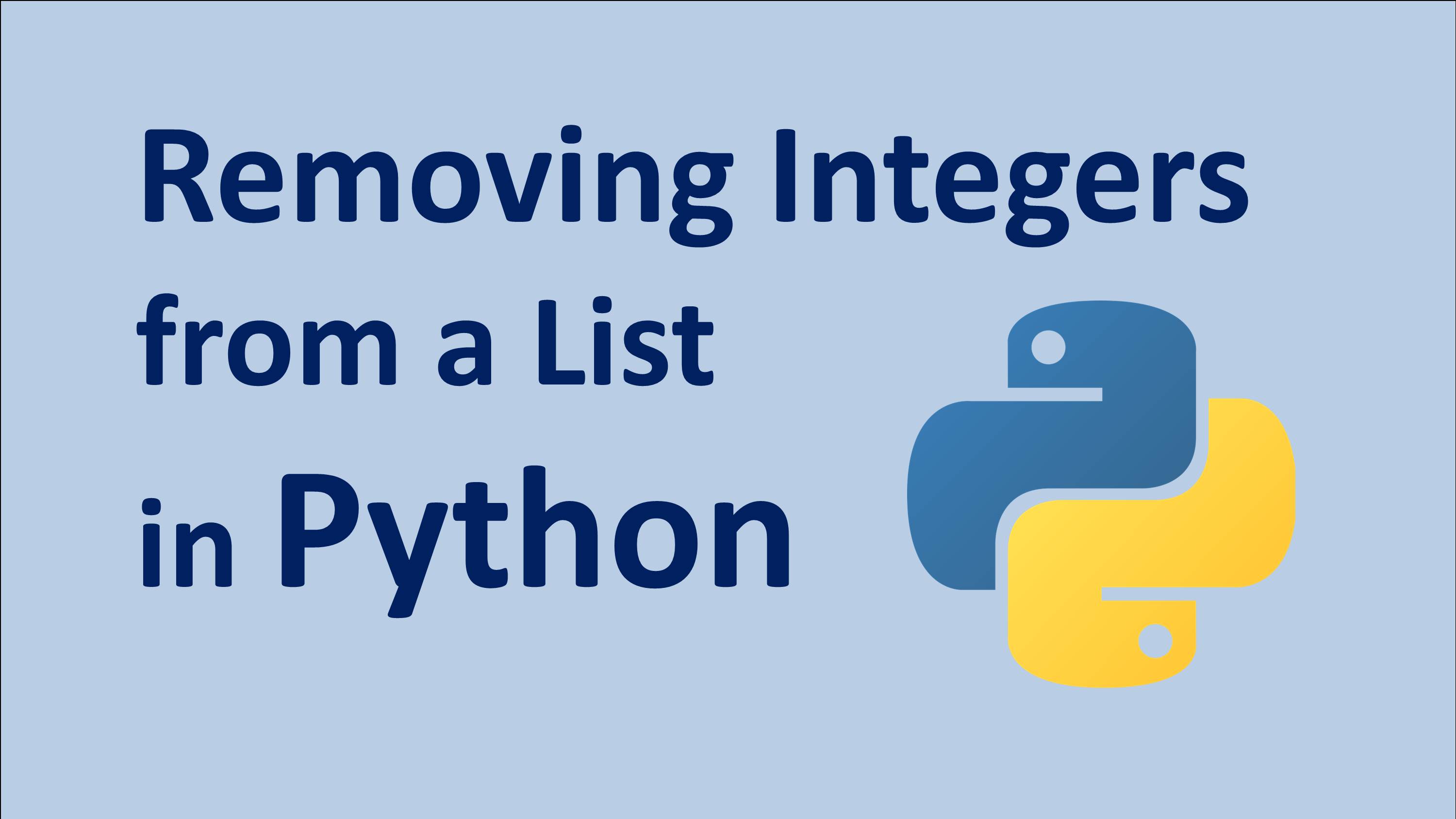
Removing integers from a List in python
When we have a list of elements which are of different data types that can include string, int, Boolean etc., and if we want to get rid of only int elements from the entire list then, python makes it simpler for us.
We have different methods to remove int values from the list. Let us discuss each method independently in more detail.
Method 1
We can remove integers or digits from the list by using python function isinstance(). By using this method, we can get items or elements which does not satisfies the function condition. Here we apply if not condition to check whether the condition is satisfied or not. Most important point to remember is this function only works only for real integers. It does not works for string type integers.
Python Code:
List_with_integers = ['1990', '1980', 'Python', 'version', '3',2000, 2020 ] no_integers = [x for x in List_with_integers if not isinstance(x, int)] print(no_integers)
Output:
[‘1990’, ‘1980’, ‘Python’, ‘version’, ‘3’]
Method 2
This method is applicable only when we have to remove the integers in the list which are in string format. It can be done by using isdigit() function in python. This method cannot able to remove real integers from the list as it produces an error stating that “int object has no attribute isdigit”.
Python Code:
List_with_integers=['1990', '1980', 'Python', 'version', '3'] no_int = [y for y in List_with_integers if not (y.isdigit() or y[0] == '-' and y[1:].isdigit())] print(no_int)
Output:
[‘Python’, ‘version’]
Method 3
This method involves try and except statements. In try statement, usually we place errors and in except, we will have an exception statement. Here, we will create an error as int datatype and except statement is taken as Value Error. In simple terms, the items or elements in the list which are int data type are ignored and print the list of elements other than integers. This method works getting rid of both real integers and integers in string format from the list, unlike the above two methods.
Python Code:
List_with_integers=['1990', '1980', 'Python', 'version', '3', 2000, 2020] def int_filter( List_with_integers ): for v in List_with_integers: try: int(v) continue # Skip these except ValueError: yield v # Keep these print(list(int_filter( List_with_integers )))
Output:
[‘Python’, ‘version’]