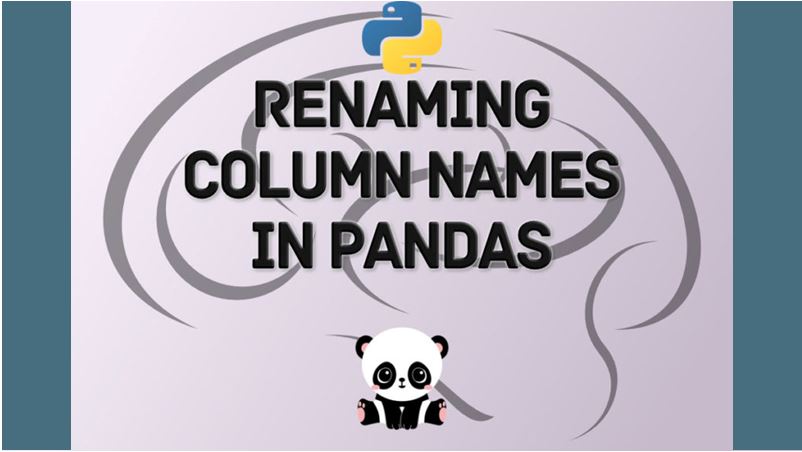
Rename Column Names in Python Pandas Dataframe
- Pandas DataFrame consists of rows and columns.
- Each row is a measurement of instance whereas column is a vector which contains data for some precise attribute/variable.
In this article let’s see how to rename column names of a given Pandas DataFrame.
Method #1:
Using rename() function.
By using the rename() function you can rename the columns in a Pandas dataframe.
# Import pandas package import pandas as pd # Define a dictionary rankings = pd.DataFrame({'Country': ['India', 'South Africa', 'England', 'New Zealand', 'Australia'], 'variable': ['Y1980', 'Y1981', 'Y1981', 'Y1980', 'Y1980'], 'value': ['21.48678', '25.22533', '22.25703', '21.46552', '21.45145']} ) # Before renaming the columns print(rankings) rankings.rename(columns = {'Country':'COUNTRY', 'variable':'VARIABLE'}, inplace = True) # After renaming the columns print("\nAfter modifying column names:\n", rankings)
Output:
Method #2:
By assigning a list of new column names
The columns can also be renamed easily by assigning a list of new names to the columns attribute of the dataframe .
# Import pandas package import pandas as pd # Define a dictionary rankings = pd.DataFrame({'Country': ['India', 'South Africa', 'England', 'New Zealand', 'Australia'], 'variable': ['Y1980', 'Y1981', 'Y1981', 'Y1980', 'Y1980'], 'value': ['21.48678', '25.22533', '22.25703', '21.46552', '21.45145']} ) # Before renaming the columns print(rankings) rankings.columns = ['COUNTRY', 'VARIABLE', 'VALUE'] # After renaming the columns print("\nAfter modifying column names:\n", rankings)
Output:
Share: