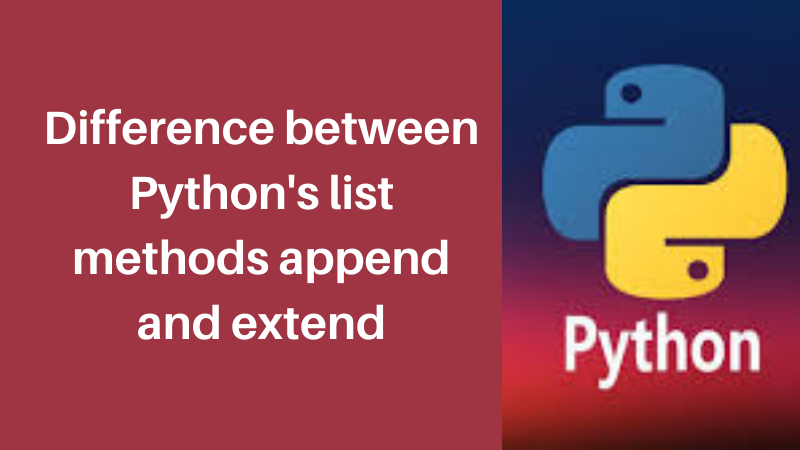
What is the difference between Python’s list methods append and extend?
List is a collection of mutable items and all of these are ordered. As we are talking about mutability, the functions extend() and append() are used to add items to the list.
Let’s see the difference between these two functions.
append():
In this method, we can only pass one element as an argument at the end of the list.
Example:
list= [1,3,5,7,9] list.append(11) print(list)
Output:
We can pass multiple elements only using another list, which gets added as a single element at the end. Look at the below example. The elements 11, 13,15 aren’t added as separate elements, rather our input list entirely is added as an element which makes it a list of lists. Look at the example below.
Example:
list= [1,3,5,7,9] list.append([11,13,15]) print(list)
Output:
extend():
We weren’t able to add multiple elements to our list using the append() method. In such situations, the extend() method comes to our rescue. Here when we pass a list of elements as an argument to this method, it concatenates our initial list with our input list, here [11,13,15], by iterating over the elements in it. Look at the following example:
Example:
list= [1,3,5,7,9] list.extend([11,13,15]) print(list) list= [1,3,5,7,9] list.extend([11]) print(list)
Output:
Note: The arguments must be in the form of a list when we are using the extend() method, even if we try to pass a single element. Whereas, we can pass a string(single element) to the append() method.
Example:
list= [1,3,5,7,9] list.extend(['Python']) print(list) list= [1,3,5,7,9] list.extend('Python') print(list)
Output:
The resulting list’s length produced after using the extend() method will be increased by the length of the iterable(list) passed to it. The append() method increases the length of the resulting list by exactly one.